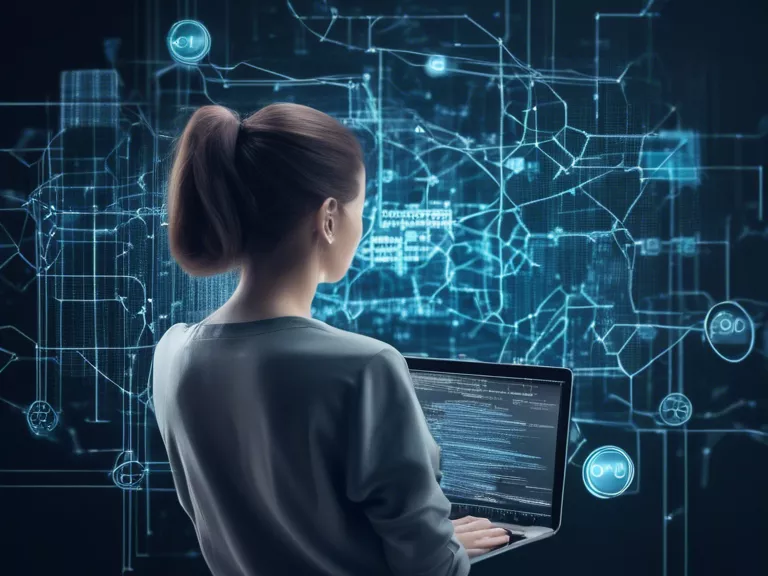
Introduction
Code optimization is a crucial aspect of software development that can greatly impact the performance and efficiency of a program. By mastering code optimization techniques, developers can create faster, more reliable, and scalable applications. In this article, we will explore some key strategies and best practices for optimizing code to achieve better performance.
Understanding Code Optimization
Code optimization is the process of improving the efficiency of a piece of code without changing its functionality. The goal is to reduce resource usage, such as memory or processing power, while maintaining or even improving the overall performance of the program. By optimizing code, developers can enhance the speed, responsiveness, and scalability of their applications.
Common Code Optimization Techniques
1. Use of Efficient Data Structures
Choosing the right data structures can significantly impact the performance of your code. Using efficient data structures, such as arrays, hash tables, or trees, can improve memory usage and reduce the time complexity of operations.
2. Minimize Loops and Nesting
Nested loops can be a major performance bottleneck in code. By minimizing the number of loops and reducing nesting levels, you can improve the overall efficiency of your code. Consider using techniques like loop unrolling or optimizing loop conditions to streamline your code.
3. Avoid Redundant Operations
Eliminating redundant operations and unnecessary calculations can help optimize code. Look for opportunities to refactor code, remove duplicate calculations, and simplify algorithms to reduce the overall workload on the system.
4. Memory Management
Proper memory management is essential for optimizing code performance. Avoid memory leaks, unnecessary allocations, and excessive memory usage by using efficient memory management techniques such as object pooling, caching, or recycling.
5. Compiler Optimization
Modern compilers offer a range of optimization options that can automatically improve code performance. Take advantage of compiler optimizations, such as inlining functions, loop unrolling, or constant propagation, to produce more efficient executable code.
Advanced Code Optimization Strategies
1. Profiling and Benchmarking
Profiling tools can help identify performance bottlenecks and hotspots in your code. By analyzing the runtime behavior of your application, you can pinpoint areas that require optimization and prioritize your efforts for maximum impact.
2. Parallelization
Utilizing parallel processing techniques, such as multi-threading or SIMD instructions, can improve the performance of your code by leveraging multiple processing units simultaneously. Parallelization can help distribute workload efficiently and reduce execution times for computationally intensive tasks.
3. Algorithm Optimization
Optimizing algorithms for efficiency can have a significant impact on code performance. Evaluate the complexity of algorithms used in your code and consider alternative approaches or optimizations to reduce time complexity and improve overall efficiency.
4. Hardware-Specific Optimization
Tailoring code optimization techniques to specific hardware architectures can yield significant performance gains. By optimizing code for CPU cache usage, instruction pipelining, or vectorization instructions, you can maximize the efficiency of your code on targeted hardware platforms.
Conclusion
Mastering code optimization techniques is essential for developing high-performance, scalable, and efficient software applications. By understanding the principles of code optimization, applying best practices, and leveraging advanced strategies, developers can create optimized code that delivers superior performance and user experience. Implementing code optimization as a core part of the software development process can lead to faster execution times, reduced resource consumption, and improved overall quality of software products.